How do you take user input in shell?
How do you take user input in shell?
It takes input from the user and assigns it to the variable. It reads only a single line from the Bash shell….Program:
- #!/bin/bash.
- # Read the user input.
- echo “Enter the user name: “
- read first_name.
- echo “The Current User Name is $first_name”
- echo.
- echo “Enter other users’names: “
- read name1 name2 name3.
How can you collect user input from a script?
So we now have 3 methods for getting input from the user: Command line arguments. Read input during script execution.
How do I prompt for input in bash?
Open a terminal and create a new file “input.sh”. Open the file and add a little code to it as below. Firstly, the echo statement is asking the user to add input value. The read statement is used to input user value, which will be saved to the variable “NAME”.
How do you read a shell script?
Reading File Content Using Script
- #!/bin/bash.
- file=’read_file.txt’
- i=1.
- while read line; do.
- #Reading each line.
- echo “Line No. $ i : $line”
- i=$((i+1))
- done < $file.
Which shell command accepts input from the user’s keyboard?
You can accept input from the keyboard and assign an input value to a user defined shell variable using read command.
Which command is used to get input from the user in database?
ACCEPT command
The ACCEPT command is used to obtain input from the user. With it, you specify a user variable and text for a prompt. The ACCEPT command displays the prompt for the user, waits for the user to respond, and assigns the user’s response to the variable.
How many ways command can get input?
In Java, there are four different ways for reading input from the user in the command line environment(console).
How do you check if a variable is equal to a string in shell?
Bash – Check If Two Strings are Equal
- Use == operator with bash if statement to check if two strings are equal.
- You can also use != to check if two string are not equal.
- You must use single space before and after the == and != operators.
How do I input multiple inputs in bash?
If you want to take multiple inputs at a time then you have to use read command with multiple variable names. In the following example, four inputs are taken in four variables by using read command.
How do you read a file into a variable in shell?
You should double-quote the variable in echo “$value” . Otherwise, the shell will perform whitespace tokenization and wildcard expansion on the value. @user2284570 Use read -r instead of just read — always, unless you specifically require the weird legacy behavior you are alluding to.
How do you use READ command?
The read command reads one line from standard input and assigns the values of each field in the input line to a shell variable using the characters in the IFS (Internal Field Separator) variable as separators.
What is EOF in a shell script?
Unix/Linux/Mac shell scripts support what is commonly called ‘Cat << EOF’ whereby an entire file can be included in a shell script and ‘restored’ to a separate file stored on the drive. In this scenario we want to be able to use a shell script to create the desired file.
How do I write a bash script?
Quick guide to writing a bash script on the Mac/Linux command-line 1. Open a new file 2. Write the shebang line: 3. Write script contents. 4. Make the script executable 5. Run the script 6. Add an input variable 7. Now run it: 8. Add an optional input variable 9. Now run it again:
What is bash prompt?
The Bash prompt is set by the environment variable PS1 (Prompt String 1), which is used for interactive shell prompts. There is also a PS2 variable, which is used when more input is required to complete a Bash command.
What is the role of shell in Unix?
In Unix, the shell is a program that interprets commands and acts as an intermediary between the user and the inner workings of the operating system.
How do you take user input in shell? It takes input from the user and assigns it to the variable. It reads only a single line from the Bash shell….Program: #!/bin/bash. # Read the user input. echo “Enter the user name: “ read first_name. echo “The Current User Name is $first_name” echo. echo “Enter other…
Recent Posts
Pages
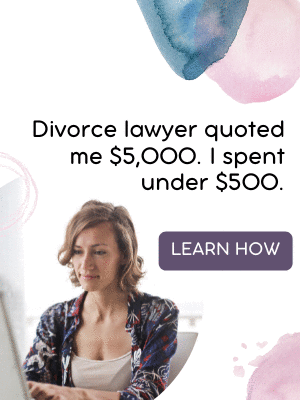
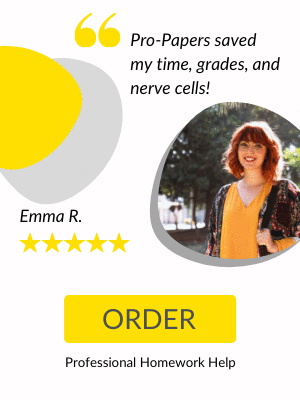