How do you find lcm and GCD in C?
How do you find lcm and GCD in C?
C Program to Find the GCD and LCM of Two Integers
- /*
- * C program to find the GCD and LCM of two integers using Euclids’ algorithm.
- #include
- void main()
- {
- int num1, num2, gcd, lcm, remainder, numerator, denominator;
- printf(“Enter two numbers\n”);
- scanf(“%d %d”, &num1, &num2);
How do you find the lcm and GCD of two numbers?
LCM(Least Common Multiple) is the smallest positive number which is divisble by both the numbers. For example lcm of 8 and 12 is 24 as 24 is divisble by both 8(8*3) and 12(12*2). HCF(Highest Common Factor)/GCD(Greatest Common Divisor) is the largest positive integer which divides each of the two numbers.
How do you find the lcm of a number in C?
Algorithm
- START.
- Step 1: Initialise two variables for num1(7) and num2(35)
- Step 2: Find and store the maximum of num1 and num2 to a separate variable, ‘max'(35)
- Step 3: If max is divisible by num1(35%7==0?) and num2(355==0?), max is the LCM(35), hence print it.
What is GCD in C programming?
The HCF or GCD of two integers is the largest integer that can exactly divide both numbers (without a remainder). There are many ways to find the greatest common divisor in C programming.
How GCD is calculated?
So, Euclid’s method for computing the greatest common divisor of two positive integers consists of replacing the larger number by the difference of the numbers, and repeating this until the two numbers are equal: that is their greatest common divisor. So gcd(48, 18) = 6.
What is the formula for finding LCM?
Also, check the LCM of two numbers to understand the steps to find the LCM of any two number easily….Formula of L.C.M.
Formulas To Calculate LCM | |
---|---|
L.C.M formula for any two numbers | L.C.M. = a×bgcd(a,b) |
What is the formula for the LCM?
Formula of L.C.M
Formulas To Calculate LCM | |
---|---|
L.C.M formula for any two numbers | L.C.M. = a×bgcd(a,b) |
LCM formula for Fractions | L.C.M. = L.C.MofNumeratorH.C.FofDenominator C . M o f N u m e r a t o r H . C . F o f D e n o m i n a t o r |
What is HCF of two numbers?
The Highest Common Factor(HCF) of two numbers is the highest possible number which divides both the numbers exactly. The highest common factor (HCF) is also called the greatest common divisor (GCD).
What is GCD example?
Ans: GCD (Greatest Common Divisor) or HCF (Highest Common Factor) of two numbers is nothing but the greatest number which divides both of them. For instance GCD of 28 and 20 is 4 and GCD of 56 and 98 is 14.
What is GCD calculator?
The GCD calculator allows you to quickly find the greatest common divisor of a set of numbers. You may enter between two and ten non-zero integers between -2147483648 and 2147483647. The numbers must be separated by commas, spaces or tabs or may be entered on separate lines.
What is LCM example?
LCM is the smallest integer which is a multiple of two or more numbers. For example, LCM of 4 and 6 is 12, and LCM of 10 and 15 is 30. One method is to factor both numbers into their primes. The LCM is the product of all primes that are common to all numbers.
How do you calculate the least common denominator?
To find the least common denominator, simply list the multiples of each denominator (multiply by 2, 3, 4, etc. out to about 6 or seven usually works) then look for the smallest number that appears in each list. Example: Suppose we wanted to add 1/5 + 1/6 + 1/15.
How do you find the greatest common denominator?
The greatest common divisor ( GCD ) of a and b is the largest number that divides both of them with no remainder. One way to find the GCD of two numbers is Euclid’s algorithm, which is based on the observation that if r is the remainder when a is divided by b, then gcd(a, b) = gcd(b, r). As a base case, we can use gcd(a, 0) = a.
Is gcd same with GCF?
Yes, there is the difference between GCF and GCD is that GCF is the greatest common factor and GCD is the greatest common denominator. A factor is a number or quantity that when multiplied with another produces a given number or expression.
What is an example of least common multiple?
Least Common Multiple. The least common multiple ( LCM ) of two or several natural numbers is the smallest positive number exactly divisible by each of the given numbers. For example, the LCM of 2 and 3 is 6, and the LCM of 6, 8, 9, 15, and 20 is 360. Least common multiples are used in adding and subtracting fractions;
How do you find lcm and GCD in C? C Program to Find the GCD and LCM of Two Integers /* * C program to find the GCD and LCM of two integers using Euclids’ algorithm. #include void main() { int num1, num2, gcd, lcm, remainder, numerator, denominator; printf(“Enter two numbers\n”); scanf(“%d %d”, &num1, &num2);…
Recent Posts
Pages
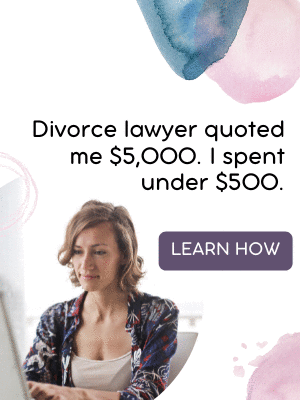
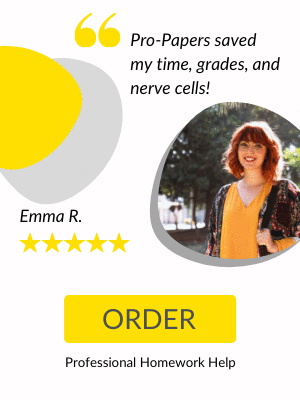