How can I get database name in PHP?
How can I get database name in PHP?
Retrieve or Fetch Data From Database in PHP
- SELECT column_name(s) FROM table_name.
- $query = mysql_query(“select * from tablename”, $connection);
- $connection = mysql_connect(“localhost”, “root”, “”);
- $db = mysql_select_db(“company”, $connection);
- $query = mysql_query(“select * from employee”, $connection);
How can I see all databases in PHP?
Use SHOW DATABASE query in place of mysql_db_list(). By using mysql_db_list() function we can get the result set and by using a pointer to this result set we can get the list of all the database. With this and using the code below we can list all the databases hosted on the mysql server.
How do I get a list of database names?
Using SQL Server Management Studio
- In Object Explorer, connect to an instance of the SQL Server Database Engine, and then expand that instance.
- To see a list of all databases on the instance, expand Databases.
What is Mysqli_select_db ()?
mysqli_select_db(mysqli $mysql , string $database ): bool. Selects the default database to be used when performing queries against the database connection. Note: This function should only be used to change the default database for the connection.
How do you create a query in PHP?
The basic steps to create MySQL database using PHP are:
- Establish a connection to MySQL server from your PHP script as described in this article.
- If the connection is successful, write a SQL query to create a database and store it in a string variable.
- Execute the query.
Is CouchDB a SQL database?
The key to remember here is that CouchDB does not work like an SQL database at all, and that best practices from the SQL world do not translate well or at all to CouchDB. This document’s “cookbook” assumes that you are familiar with the CouchDB basics such as creating and updating databases and documents.
What is mysql_query?
mysql_query() sends a query to the currently active database on the server that’s associated with the specified link identifier. For other type of SQL statements, mysql_query() returns TRUE on success and FALSE on error. A non-FALSE return value means that the query was legal and could be executed by the server.
What is DB PHP?
» Description. DB is a database abstraction layer providing: * an OO-style query API. * portability features that make programs written for one DBMS work with other DBMS’s. * a DSN (data source name) format for specifying database servers.
How do I run PHP code locally?
To locally run a PHP Script:
- Click the arrow next to the Run button. on the toolbar and select Run Configurations -or- go to Run | Run Configurations. A Run dialog will open.
- Double-click the PHP Script option to create a new run configuration.
How to get username from database in PHP?
According to your query, you’re trying to select a username from the database which is equal to $connect = mysql_connect (“$server”, “$dbuser”) – this doesn’t make sense at-all. Note: this is a very basic script.
When to use MySQL _ db _ name in PHP?
mysql_db_name. (PHP 4, PHP 5) mysql_db_name — Retrieves database name from the call to mysql_list_dbs () Warning. This extension was deprecated in PHP 5.5.0, and it was removed in PHP 7.0.0. Instead, the MySQLi or PDO_MySQL extension should be used.
How to get column names from table in PHP?
In the example code snippet, we will show you how to get and show the column names from a table using PHP and MySQL. The following query will find the column names from a table in MySQL and returns all the columns of the specified table. Use TABLE_SCHEMA to select the columns of a table from a specific database.
How to get list of databases in MySQL?
At the MySQL prompt, SHOW DATABASES does what you want. You can run this command as a query from PDO or the native PHP MySQL library and read the returned rows. Pretend it is a normal select. You will only see the databases that the account used to connected to MySQL can see.
How can I get database name in PHP? Retrieve or Fetch Data From Database in PHP SELECT column_name(s) FROM table_name. $query = mysql_query(“select * from tablename”, $connection); $connection = mysql_connect(“localhost”, “root”, “”); $db = mysql_select_db(“company”, $connection); $query = mysql_query(“select * from employee”, $connection); How can I see all databases in PHP? Use SHOW DATABASE query…
Recent Posts
Pages
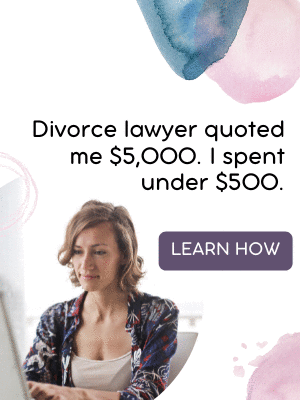
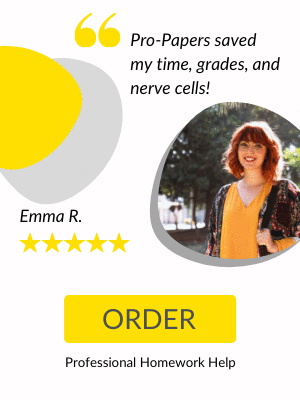